Using Cloudflare Workers to make API Calls
Introduction
It's been a common topic around the Riot Games API Community that CORS has prevented client side calls to the API.
There is a guide that Tux made for v3 APIs with AWS Lambda functions, but what if we wanted something more up to date and flexible that we don't need to constantly update?
I've made a script to use any endpoint and help cache responses to help alleviate rate limiting concerns, although if you're requesting a lot of matches through the function you could still run into issues on rate limits.
Caching data on Lambda is more complex/pricey, but this script could be modified to be used on Lambda as well, I just prefer it less.
The downside of using these functions is that anybody can technically use your endpoints and use your function calls/Riot API Limits, if this is a concern I'd recommend you create a back-end to your app. This could be in anything like Node.js, Python, Golang, Java, or whatever today's flavor of the day is.
Getting Started
With that data out of the way, let's get started.
You will need a Cloudflare account. You can sign up for one at https://dash.cloudflare.com/sign-up
For this example we will be using the Free plan of Cloudflare Workers, you can make up to 100,000 requests per day, if you need above that amount you should probably consider a backend.
To get started go to https://workers.cloudflare.com/
Sign up if you haven't already, choose a domain name that matches your project, then choose the plan that fits you.
Once done create a service, name it something that makes sense. For this we are using the name example, keep Introduction chosen we are going to replace the code anyways.
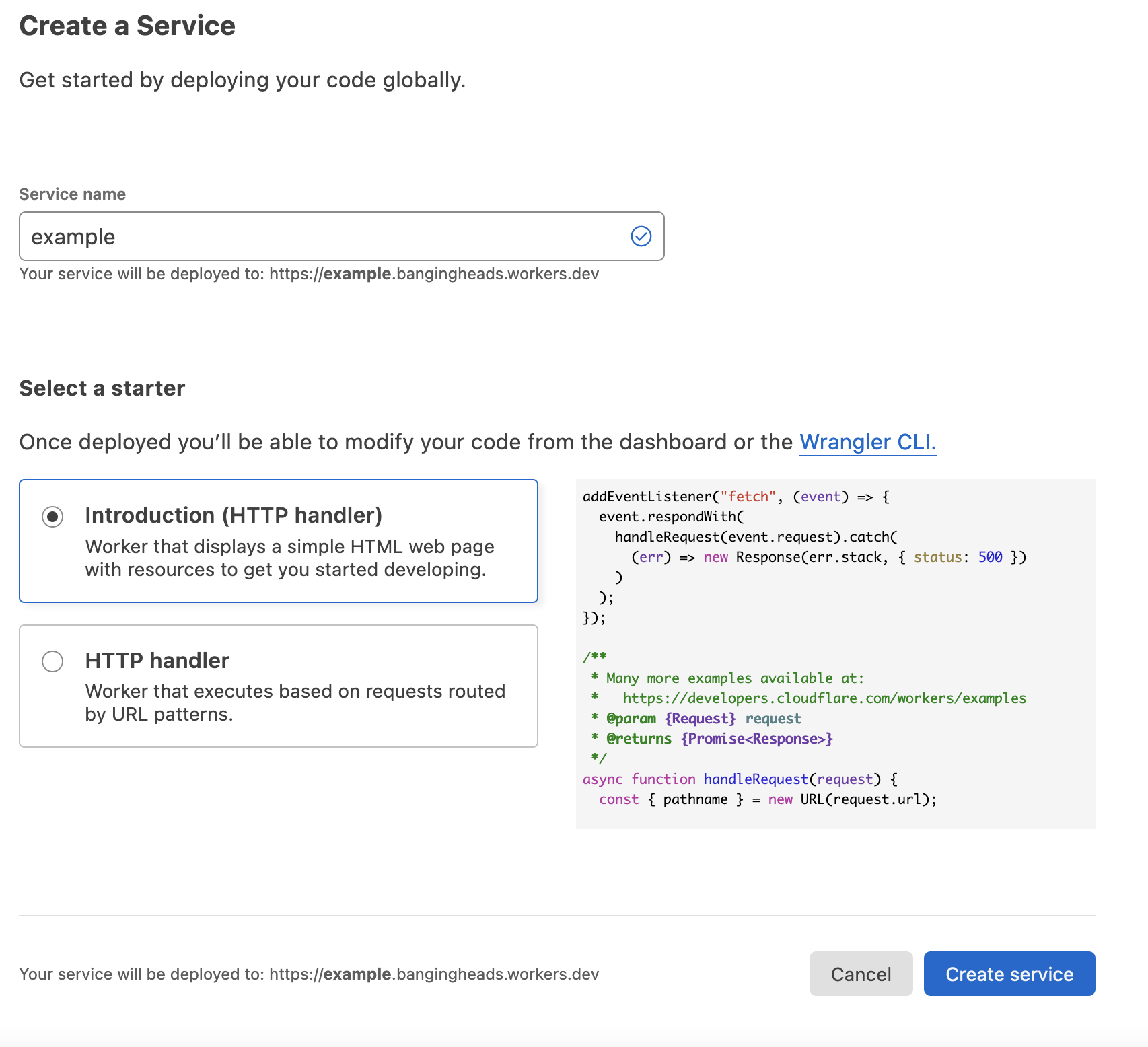
Once you got your service up and going, choose Quick Edit.
We are going to use code from a gist I've made so that there is minimal effort in the coding needed.
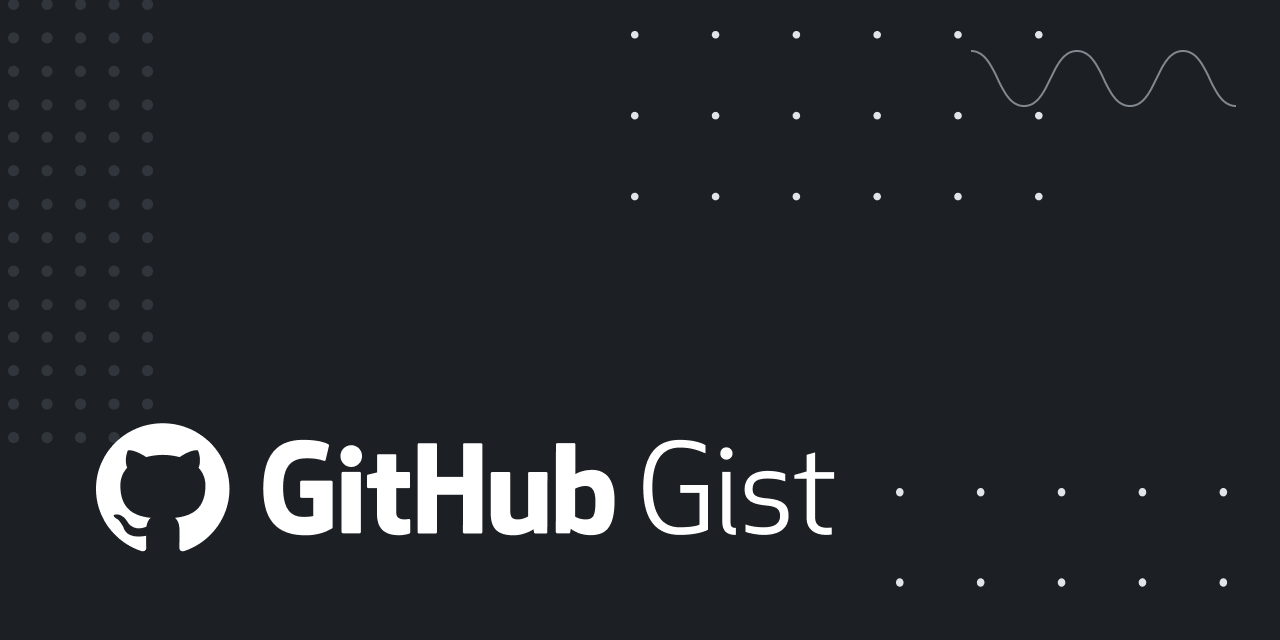
Go ahead and copy and paste the code into your worker, replacing any code it originally gave you in its example.
We will need to change our constants at the top of the script to match our needs.
API_KEY: Your Riot Games API key, this can be a developer, personal, or production key.
API_ENDPOINT: Unless you have a reason you don't need to change this
CACHE_TIME: This by default is 10 minutes, this means once you make an API call it won't make the same call for 10 minutes, if you're trying to do something like fetch a current match or the latest match you may want to lower this.
ALLOWED_ORIGINS: Change this to your domain name, if you're using www include www, if not don't. If you're using both include both. Use a * if you'd like to allow all domains but this will allow anybody to use your workers.
Once you have those all set you're ready to make an API call through, go ahead Save and Deploy!
Examples
Example 1: Path Parameters
The endpoint parameter will be exactly as it shows on the Riot Game Developer portal, in our example we are using the Summoner-V4 endpoint by-name.
This endpoint is /lol/summoner/v4/summoners/by-name/{summonerName}.
Since this is a v4 league endpoint we will use a platform region of na1.
The way this script is setup is any path parameters like summonerName here we will append as an additional GET parameter. You also can replace it in the endpoint as well if it makes more sense for you.
So for this request we will use:
https://example.bangingheads.workers.dev/api/?region=na1&endpoint=/lol/summoner/v4/summoners/by-name/{summonerName}&summonerName=Headbang
Now obviously you will need to change this to your domain name to test it.
We should get our response of the Summoner-V4 JSON.
Example 2: Additional GET Parameters
This also works for endpoints that have additional get parameters that we can add to the end of the URL.
For example Match-V5 has a count parameter, we are setting count to 100 here.
Our endpoint is /lol/match/v5/matches/by-puuid/{puuid}/ids
Our region is americas
Our example puuid is f83au5bQ3XCRxvP3_xAEcki5wnHZ22ZhSkbp5wRpg0j5W-pJnPL_C1q3QVdWBV9xF8g_5ADYduuEZg
This builds our URL into:
https://example.bangingheads.workers.dev/api/?region=americas&endpoint=/lol/match/v5/matches/by-puuid/{puuid}/ids&puuid=f83au5bQ3XCRxvP3_xAEcki5wnHZ22ZhSkbp5wRpg0j5W-pJnPL_C1q3QVdWBV9xF8g_5ADYduuEZg&count=100
Now that you understand the basics, feel free to make any modifications to the script as you find are needed, or feel free to ping me in the Riot API/Hextech Docs discord @BangingHeads with any questions.